JRuby/Gradle
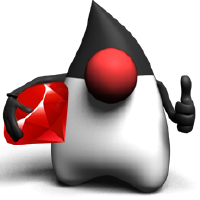
JRuby/Gradle is a collection of Gradle plugins which make it easy to build, test, manage and package Ruby applications. By combining the portability of JRuby with Gradle’s excellent task and dependency management, JRuby/Gradle provides high quality build tooling for Ruby and Java developers alike.
Plugins available:
Quick Start
Running some Ruby
The simplest example is a hello-world from Gradle, using JRuby/Gradle we can execute a Ruby script, which has Ruby-based dependencies:
build.gradle (full source)
buildscript {
repositories { jcenter() }
dependencies {
/* Replace "%%VERSION%%" with the version of JRuby/Gradle you wish to
* use if you want to use this build.gradle outside of gradleTest
*/
classpath 'com.github.jruby-gradle:jruby-gradle-plugin:%%VERSION%%'
}
}
apply plugin: "com.github.jruby-gradle.base"
import com.github.jrubygradle.JRubyExec
dependencies {
/* Using the built-in `jrubyExec` configuration to describe the
* dependencies our JRubyExec-based tasks will need
*/
jrubyExec "rubygems:colorize:0.7.7+"
}
task printSomePrettyOutputPlease(type: JRubyExec) {
description "Execute our nice local print-script.rb"
script "${projectDir}/print-script.rb"
}
print-script.rb (full source)
require 'colorize'
puts "-" * 20
puts "Ruby version: #{RUBY_VERSION}"
puts "Ruby platform: #{RUBY_PLATFORM}"
puts "-" * 20
puts "Roses are red".red
puts "Violets are blue".blue
puts "I can use JRuby/Gradle".green
puts "And now you can too!".yellow
Executing ./gradlew printSomePrettyOutputPlease
results in the following:
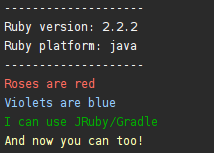
Packaging some Ruby
build.gradle (full source)
buildscript {
repositories { jcenter() }
dependencies {
/* here to make sure that our dependencies get loaded in properly under
* GradleTest, this is NOT needed by end-users
*/
classpath 'com.github.jengelman.gradle.plugins:shadow:[1.2.2,2.0)'
/* Replace "%%VERSION%%" with the version of JRuby/Gradle you wish to
* use if you want to use this build.gradle outside of gradleTest
*/
classpath 'com.github.jruby-gradle:jruby-gradle-plugin:%%VERSION%%'
classpath 'com.github.jruby-gradle:jruby-gradle-jar-plugin:%%VERSION%%'
}
}
apply plugin: "com.github.jruby-gradle.jar"
repositories { jcenter() }
dependencies {
/* Using the built-in `jrubyJar` configuration to describe the
* dependencies our jrubyJar task will need, so the gem is properly
* included in the resulting .jar file
*/
jrubyJar "rubygems:colorize:0.7.7+"
jrubyJar 'org.slf4j:slf4j-simple:1.7.12'
}
jrubyJar {
/* We want to use this Ruby script as our start point when the jar executes
*/
initScript "${projectDir}/entrypoint.rb"
}
entrypoint.rb (full source)
require 'colorize'
java_import 'org.slf4j.Logger'
java_import 'org.slf4j.LoggerFactory'
logger = LoggerFactory.getLogger('demo')
puts "-" * 20
logger.info "Ruby version: #{RUBY_VERSION}"
logger.info "Ruby platform: #{RUBY_PLATFORM}"
logger.info "Current file: #{__FILE__}"
puts "-" * 20
puts "Roses are red".red
puts "Violets are blue".blue
puts "I can use JRuby/Gradle".green
puts "And now you can too!".yellow
Executing ./gradlew jrubyJar
will build a .jar
file inside of the
build/libs
directory which can then be invoked:
% java -jar build/libs/self-executing-jar-jruby.jar
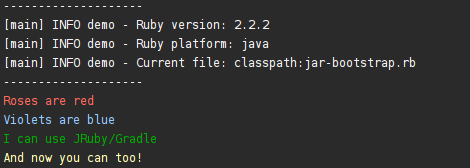